
Are you looking for information or an example of how to implement picking up items in Unity?
Look no further! In this post, you will find a simple solution for that.
We will make the first-person character with movement and grabbing scripts, and we will make another one for objects that we would like to pick up.
Let’s see how we can do that! ?
Character ?♂️
First of all, we will need a character that can move around in the scene.
We will build that character from a capsule, and we will move our primary camera to be a child of this object.

Next, we have to create a movement script. We will use a CharacterController component with our simple movement script.
using UnityEngine; /// <summary> /// Simple player movement. /// </summary> [RequireComponent(typeof(CharacterController))] public class SimplePlayerMovement : MonoBehaviour { [Header("Character")] // Reference to the character controller [SerializeField] private CharacterController character; // Character movement speed. [SerializeField] private float moveSpeed = 4; [Header("Camera")] // Reference to the character camera. [SerializeField] private Camera characterCamera; // Camera movement speed. [SerializeField] private float camSpeed = 40; /// <summary> /// Method called at the start. /// </summary> private void Start() { // Lock and hide cursor Cursor.lockState = CursorLockMode.Locked; Cursor.visible = false; } /// <summary> /// Method called every frame. /// </summary> private void Update() { // Get player input for character movement var v = Input.GetAxis("Vertical"); var h = Input.GetAxis("Horizontal"); // Create move vector and rotate it var move = new Vector3(h, 0, v); move = character.transform.rotation * move; // If length of move vector is bigger than 1, normalize it. if (move.magnitude > 1) move = move.normalized; // Move character character.SimpleMove(move * moveSpeed); // Get player mouse input for camera and character rotation var mx = Input.GetAxisRaw("Mouse X"); var my = Input.GetAxisRaw("Mouse Y"); // Rotate character with mouse X value character.transform.Rotate(Vector3.up, mx * camSpeed); // Get camera rotation on X axis var currentRotationX = characterCamera.transform.localEulerAngles.x; currentRotationX += my * camSpeed; // Limiting camera movement to (-60) - (60) degrees on X axis. if (currentRotationX < 180) { currentRotationX = Mathf.Min(currentRotationX, 60); } else if (currentRotationX > 180) { currentRotationX = Mathf.Max(currentRotationX, 300); } // Assign new camera rotation characterCamera.transform.localEulerAngles = new Vector3(currentRotationX, 0, 0); } }
With this script ready, now we can attach it to the player object and assign references.

Grabbing system ?
Creating a grabbing system is not that hard to begin with. We basically need only two classes. One, with we will attach to the player, and the second one, which we will attach to the pickable objects.
But before we can implement them, we need to create a slot where we will hold our picked objects. You only need to add an empty object (or cube) under the player camera so we can see that we have something in our “hand”.

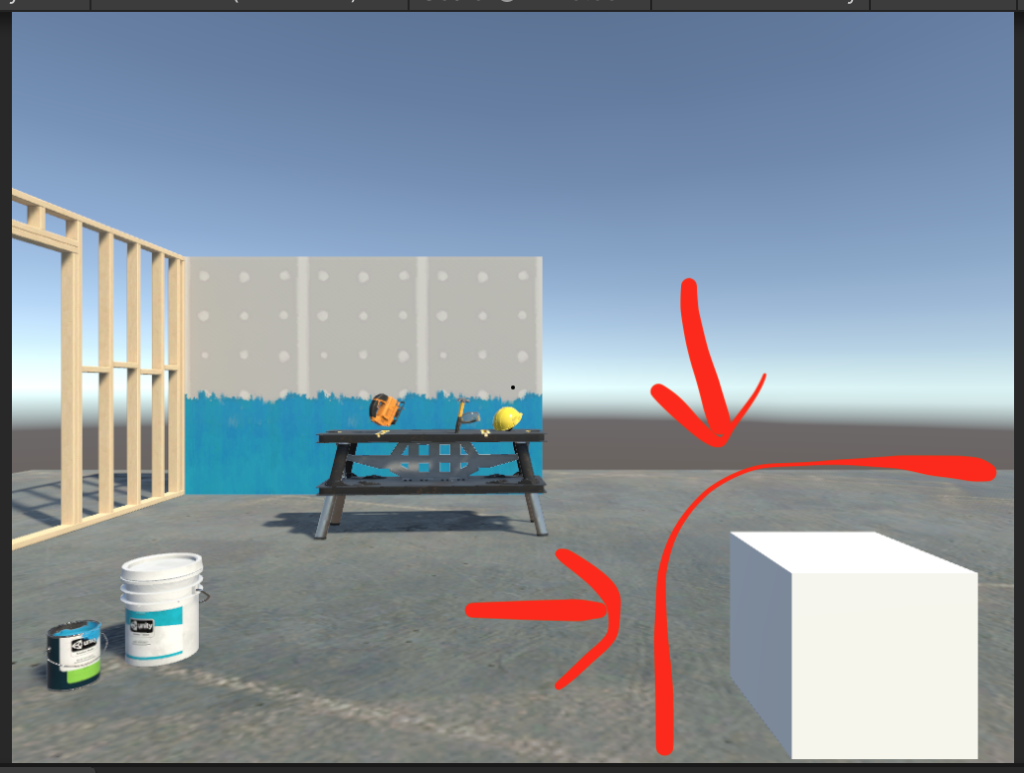
Making object pickable ?
Now it’s time to create new classes for our system! Let’s start with PickableItem first, as it will be a really short script.
using UnityEngine; /// <summary> /// Attach this class to make object pickable. /// </summary> [RequireComponent(typeof(Rigidbody))] public class PickableItem : MonoBehaviour { // Reference to the rigidbody private Rigidbody rb; public Rigidbody Rb => rb; /// <summary> /// Method called on initialization. /// </summary> private void Awake() { // Get reference to the rigidbody rb = GetComponent<Rigidbody>(); } }
With this class, we can make object pickable. I would recommend creating a “parent” game object with this component, as it will be easier to rotate and position it in a slot.


Now, you just need to attach our component to the parent and a Mesh Collider to the model itself with “Convex” option enabled.


Let’s grab some items! ?
The second script of our system will be attached to the player and will be responsible for handling picking up items and dropping them onto the floor.
Let’s create SimpleGrabSystem class!
using UnityEngine; /// <summary> /// Simple example of Grabbing system. /// </summary> public class SimpleGrabSystem : MonoBehaviour { // Reference to the character camera. [SerializeField] private Camera characterCamera; // Reference to the slot for holding picked item. [SerializeField] private Transform slot; // Reference to the currently held item. private PickableItem pickedItem; /// <summary> /// Method called very frame. /// </summary> private void Update() { // Execute logic only on button pressed if (Input.GetButtonDown("Fire1")) { // Check if player picked some item already if (pickedItem) { // If yes, drop picked item DropItem(pickedItem); } else { // If no, try to pick item in front of the player // Create ray from center of the screen var ray = characterCamera.ViewportPointToRay(Vector3.one * 0.5f); RaycastHit hit; // Shot ray to find object to pick if (Physics.Raycast(ray, out hit, 1.5f)) { // Check if object is pickable var pickable = hit.transform.GetComponent<PickableItem>(); // If object has PickableItem class if (pickable) { // Pick it PickItem(pickable); } } } } } /// <summary> /// Method for picking up item. /// </summary> /// <param name="item">Item.</param> private void PickItem(PickableItem item) { // Assign reference pickedItem = item; // Disable rigidbody and reset velocities item.Rb.isKinematic = true; item.Rb.velocity = Vector3.zero; item.Rb.angularVelocity = Vector3.zero; // Set Slot as a parent item.transform.SetParent(slot); // Reset position and rotation item.transform.localPosition = Vector3.zero; item.transform.localEulerAngles = Vector3.zero; } /// <summary> /// Method for dropping item. /// </summary> /// <param name="item">Item.</param> private void DropItem(PickableItem item) { // Remove reference pickedItem = null; // Remove parent item.transform.SetParent(null); // Enable rigidbody item.Rb.isKinematic = false; // Add force to throw item a little bit item.Rb.AddForce(item.transform.forward * 2, ForceMode.VelocityChange); } }
Great! The only thing left is to attach this class to the player!

The result ?
So let’s finally test that thing!

And as you can see, we created a simple solution for picking and dropping items in Unity.
If you found that helpful, share what project you are working on in the comment section below! I would love to know! ?
You can check the whole project at my public repository. ?
And if you are interested in getting emails when I release a new post, sign up for the newsletter!
Hope to see you next time! ?
hey, this looks cool,,, but I don’t know how to implement it…. like, I created the code and all… but , how can I apply it to a background scene.
For me, I want a simple soccer player to move a ball by having the ball follow him.
So i have a gun and i want it disabled when its not picked up but want it set active when picked up could somebody help me
how do i use the rigidbobby
Didn’t work, i cant pick up the object and no errors show up so idk what it is, it does just not pick up
Maybe you missed some steps? Do you have colliders and rigidbodies? Download the repository (link at the end of the post) and see the differences.
is there a way we can pick up a item and it destroy the item?
Sure, there is Destroy() method. Pass the gameObject there and the item will be destroyed.
Well, After going over 7 or 8 tutors on you tube today, trying to find someone who could teach me how to make a pick up and drop script on the same button, I finally found this page, and low and behold it worked, then I changed the ray distance(longer) and also the mouse Y was inverted to multiplied that by -1, so down was down, and it all stopped working, cant pick anything up, so I figured maybe the -1 was shooting the ray out the back, so changed it back to how it is on this page, and… Read more »
Can I use this script on android game?
yeah just make android controls
how can i use this script on androidgame with buttons? I wanna that when I touch button , i can pick up item and other button which named button when i touch , i can drop it. What difference i do it in this script??
Could we add velocity to the gameobject when it is dropped?
Hi patryk, great tutorial. I’ve pretty much managed to implement this but for one small bug I can’t seem to figure out. After I pick up the object it goes to the slot and then seems to shift out of the view , so it’s like it’s attached to the slot but with an offset
Hi, your scripts are great, i can make it work on my project. But one little thing, the grabbed item can pass through kinematic object.
When you set the flag “isKinematic” in rigidbody to true you are responsible for handling the physic behavior of the object. It was perfect for this example but you can change that and leave it as false, but you would need to create a joint that will hold grabbed object in your hand.
hey I was recreating this script, and when i was done the items changed shape when i picked them up. Does anyone know how to fix this?
Hey, great tutorial, Ive been looking for ages for a system like this, but I have a question – how would I make this work on a third person game? I tried changing the camera references so the script references an empty game object attached to the player that would act as the origin of the ray, but nothing happens.
so i did all the steps and my block only disapears it deos not go to my hand
uhh when i look at a certain angle when im holding the object it puts the object to shadows only and dissappears but if i put it back to the same position it comes back
this code is very good and i love it i works so well
when i pick up an item the scale changes how do i fix that